I've done some projects in the past where we created
Scalable Vector Graphics. The nice thing about SVG is that you can scale the images without any quality loss. Back then, the only way to render SVG in the browser was by installing a plugin from Adobe. Now most browsers are able to render them without any plugins.
So how do you get started? This little tutorial assumes you have some basic
Cocoon knowledge
Create a SVG template with the SVG editor of your choice.Some suggestions:
Replace the hardcoded values which should become parameters like this: ${logotext}
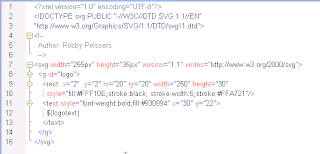
Below you will find some relevant sitemap.xmap snippets which i configured to create a dynamic image.

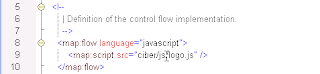
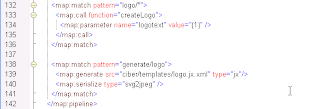
If we take a quick look at line 138, you see that when we invoke the url wich matches "generate/logo", the template "ciber/templates/logo.jx.xml" is read, the ${} values are replaced by their corresponding values if present and the svg-template is serialized to jpeg.
We only need some way to fill that missing ${logotext} parameter. Cocoon offers flowscript to the rescue. Flowscript is plain javascript in which you can write the controller which takes care of building the model which will be passed on to the view. (Model-View-Controller).
Our controller will use a sitemap parameter to fill in the missing ${logotext}. In line 132, you see a match pattern "logo/*" where * is our first and only {1} parameter which we pass on to the flowscript function "createLogo" on line 134.
Let's take a quick look at our flowscript (logo.js)
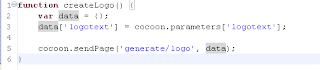
When you invoke following URL:
"http://localhost:8888/cocoonDemo/logo/Creating SVG with Cocoon" the sitemap matches this on line 132. It call's the function createLogo() and passes it the sitemap parameter 'logotext' with value 'Creating SVG with Cocoon'.
On lines 2 and 3 we build a javascript object 'data' with a variable named logotext and pass it the value of the sitemap-parameter with the same name. On line 5 our controller tells cocoon to render a page by invoking the
match pattern "generate/logo" and pass it our constructed model 'data'.
Now the sitemap matches line 138, but this time it does have a model containing a value for 'logotext'. Let's take a look at the end result.
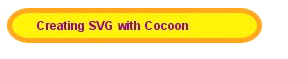
This demo shows you the most basic (simple) case to create dynamic SVG. More difficult use cases are when you read in your data from a database or XML files and use this data in transformations to construct SVG.
Cheers,
Robby